Python Qt Slot Decorator
The following are 20 code examples for showing how to use PySide2.QtCore.Slot.These examples are extracted from open source projects. You can vote up the ones you like or vote down the ones you don't like, and go to the original project or source file by following the links above each example.
Decorators are a significant part of Python. In simple words: they arefunctions which modify the functionality of other functions. They helpto make our code shorter and more Pythonic. Most beginners do notknow where to use them so I am going to share some areas wheredecorators can make your code more concise.
First, let’s discuss how to write your own decorator.
Qt’s widgets have many pre-defined slots, but it is common practice to subclass widgets and add your own slots so that you can handle the signals that you are interested in. The signals and slots mechanism is type safe: The signature of a signal must match the signature of the receiving slot. Adding QtCore.Slot decorator to base class function influences which thread executes slot in a derived class. Automatically Connecting Slots by Name. The code in attachment to replace connectSlotsByName has been working great for me. I'm replacing more and more QObject.connect -like code with it (I could not use the connectSlotsByName from Qt because it binds callbacks twice (and yes, even if I did decorate my callbacks) and most importantly because my callbacks are not on the same object.
It is perhaps one of the most difficult concepts to grasp. We will takeit one step at a time so that you can fully understand it.
7.1. Everything in Python is an object:¶
First of all let’s understand functions in Python:
7.2. Defining functions within functions:¶
So those are the basics when it comes to functions. Let’s take yourknowledge one step further. In Python we can define functions insideother functions:
So now we know that we can define functions in other functions. Inother words: we can make nested functions. Now you need to learn onemore thing, that functions can return functions too.
7.3. Returning functions from within functions:¶
It is not necessary to execute a function within another function, wecan return it as an output as well:
Just take a look at the code again. In the if/else
clause we arereturning greet
and welcome
, not greet()
and welcome()
.Why is that? It’s because when you put a pair of parentheses after it, thefunction gets executed; whereas if you don’t put parenthesis after it,then it can be passed around and can be assigned to other variableswithout executing it. Did you get it? Let me explain it in a little bitmore detail. When we write a=hi()
, hi()
gets executed andbecause the name is yasoob by default, the function greet
is returned.If we change the statement to a=hi(name='ali')
then the welcome
function will be returned. We can also do print hi()()
which outputsnow you are in the greet() function.
7.4. Giving a function as an argument to another function:¶
Now you have all the required knowledge to learn what decorators reallyare. Decorators let you execute code before and after a function.
7.5. Writing your first decorator:¶
In the last example we actually made a decorator! Let’s modify theprevious decorator and make a little bit more usable program:
Did you get it? We just applied the previously learned principles. Thisis exactly what the decorators do in Python! They wrap a function andmodify its behaviour in one way or another. Now you might bewondering why we did not use the @ anywhere in our code? That is just ashort way of making up a decorated function. Here is how we could haverun the previous code sample using @.
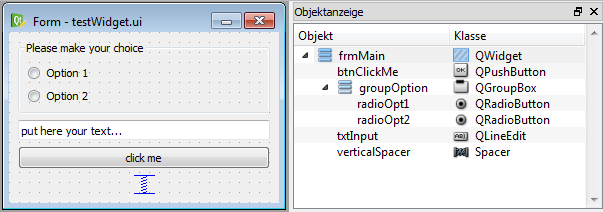
I hope you now have a basic understanding of how decorators work inPython. Now there is one problem with our code. If we run:
That’s not what we expected! Its name is“a_function_requiring_decoration”. Well, our function was replaced bywrapTheFunction. It overrode the name and docstring of our function.Luckily, Python provides us a simple function to solve this problem andthat is functools.wraps
. Let’s modify our previous example to usefunctools.wraps
:
Now that is much better. Let’s move on and learn some use-cases ofdecorators.
Blueprint:
Note: @wraps
takes a function to be decorated and adds thefunctionality of copying over the function name, docstring, argumentslist, etc. This allows us to access the pre-decorated function’s propertiesin the decorator.
7.5.1. Use-cases:¶
Now let’s take a look at the areas where decorators really shine andtheir usage makes something really easy to manage.
7.5.2. Authorization¶
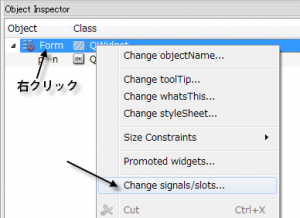
Decorators can help to check whether someone is authorized to use anendpoint in a web application. They are extensively used in Flask webframework and Django. Here is an example to employ decorator basedauthentication:
Example :
7.5.3. Logging¶
Logging is another area where the decorators shine. Here is an example:
I am sure you are already thinking about some clever uses of decorators.
7.6. Decorators with Arguments¶

Come to think of it, isn’t @wraps
also a decorator? But, it takes anargument like any normal function can do. So, why can’t we do that too?
This is because when you use the @my_decorator
syntax, you areapplying a wrapper function with a single function as a parameter.Remember, everything in Python is an object, and this includesfunctions! With that in mind, we can write a function that returnsa wrapper function.
7.6.1. Nesting a Decorator Within a Function¶
Let’s go back to our logging example, and create a wrapper which letsus specify a logfile to output to.
7.6.2. Decorator Classes¶
Now we have our logit decorator in production, but when some partsof our application are considered critical, failure might besomething that needs more immediate attention. Let’s say sometimesyou want to just log to a file. Other times you want an email sent,so the problem is brought to your attention, and still keep a logfor your own records. This is a case for using inheritence, butso far we’ve only seen functions being used to build decorators.
Luckily, classes can also be used to build decorators. So, let’srebuild logit as a class instead of a function.
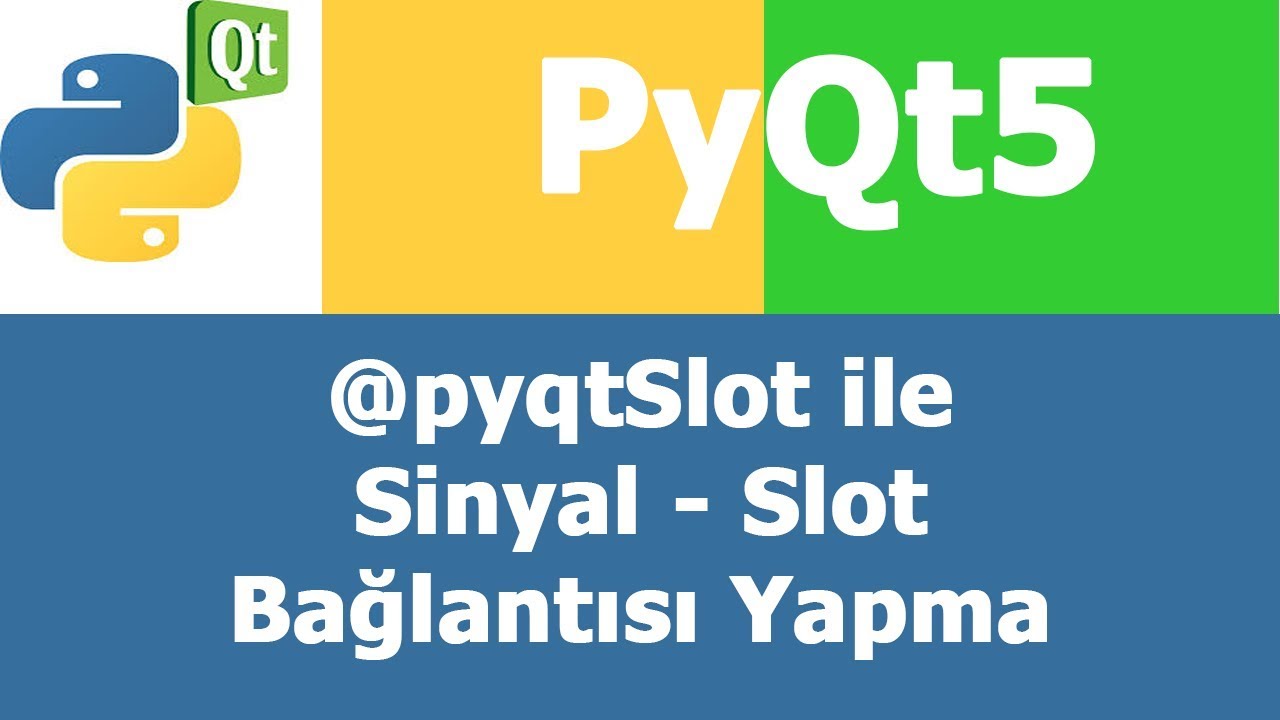
This implementation has an additional advantage of being much cleaner thanthe nested function approach, and wrapping a function still will usethe same syntax as before:
Python Qt Slot Decorator Machine
Now, let’s subclass logit to add email functionality (though this topicwill not be covered here).
Python Qt Designer Slot
From here, @email_logit
works just like @logit
but sends an emailto the admin in addition to logging.